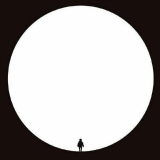
Gagandeep Singh
== vs === 🔗When working with JavaScript, you may come across the operators == and ===. These operators are used for comparing values in JavaScript, but they work in slightly different ways. In this blog post, we’ll explore the differences between == and === in JavaScript and when you should use each one.
The == operator is known as the equality operator. It is used to compare the values of two operands, and if they are equal, it returns true.
Arrays 🔗Like any other programming language, arrays in Go are used to group elements of same type and has a fixed length together. Whenever an array is declared or initialized the type of array has to be defined. Type of elements and the length are both used to defined the type of the array. For example, the array below can store string values and it’s length is 4, so its type is [4]string.
#Why type=“module” is used in the <script> element?
Rather than writing out whole Javascript application in one big file, it can be broken down into multiple files called modules. Modules are just .js files which can be imported into other files and has some exported code (functions or variables).
export const USER_ACCESS_TYPES = { admin: "full access", contributor: "read, write access", user: "read access", }; export const getUserAccessType = (userType) => { return USER_ACCESS_TYPES[userType]; }; These values can be imported in other script files and used.
Types đź”—A type helps in defining the shape or structure of the javascript value. A type can consist of a single property or method or can have combination of multiple properties and methods.
For example, the type of variables below can be inferred by typescript by checking the values assigned to them.
let id = 123; //number let name = "Gagandeep Singh"; //string let skills = ["typescript", "react"]; // string[] But in case of an object or complex data structure it’s good to define a type beforehand.
Union Types đź”—Multiple types can be defined on a value by using the | symbol. When a value is expected to have one of many known types, then using union type is the most suitable option (rather than any or unknown).
type value = number | boolean; Here value can be either number or boolean.
We can also combine custom type using the union operator.
interface house { hasDoor: boolean; address: string; } interface building { hasLift: boolean; address: string; } type houseOrBuilding = house | building const hb: houseOrBuilding In above case hb can only access members that are common to all types in the union.